Serialization is the process of converting complex objects into a data format that can be easily stored or sent across a network connection. In JavaScript, serialization and deserialization of objects can be achieved by using JSON.stringify()
and JSON.parse()
.
Example
Coerce object into class typescript; typescript deserialize json with only strings to proper types; typescript from json to object; json parse to type typescript; json parse typescript cast to interface; typescript json object data type; typescript json; parse json to model typescript; parsemodel typescript; should i convert json to class. I started working with TypeScript about two years ago. Most of the time I read a JSON object from a remote REST server. This JSON object has all the properties of a TypeScript class. There is a question always buzz my head: How do I cast/parse the received JSON object to an instance of a corresponding class?
Things start to get complicated when you are using TypeScript
or ES6
and the response from the server doesn’t really match your client data structure. In this case you have an extra step of copying the properties from the parsed json response into your custom model object. When you want to send the data back to the server you have to copy the properties again into their original format.
Typescript library for deserializing one object type to another. Table of contents. Installation; Description; API Documentation; Installation. Npm install ts-deserializer -save. Ts-deserializer can be used to deserialize data from one class to another. Another common use case is deserializing data that should be of a given type. Serialize and deserialize javascript object, preserve your object model. Persistance and serialization in javascript and nodejs. Serialijse is an simple persistence framework for JavaScript that overcomes two main limitation of JSON persistence: serialijse deals well with cyclic objects. Serialijse preserve object class upon deserialization. The parsed JSON string is loaded to an object of specified TypeScript class. And we can access the properties of class object just like we access the elements of JSON object, using dot operator. TutorialKart com Conclusion. In this TypeScript Tutorial, we learned how to parse a JSON string to a JSON object or to a custom TypeScript.
Example
Some time ago I have started using TypeScript for most of my projects. One of those projects had a very different data structure between the server and the client. After getting very frustated with writing serialization and deserialization methods for my data models, I came up with the ideea of ts-serializer. ts-serializer
is a collection of typescript decorators and helper classes that allows the developer to easily serialize and deserialize complex objects to and from JSON objects.
Installation
Using NPM
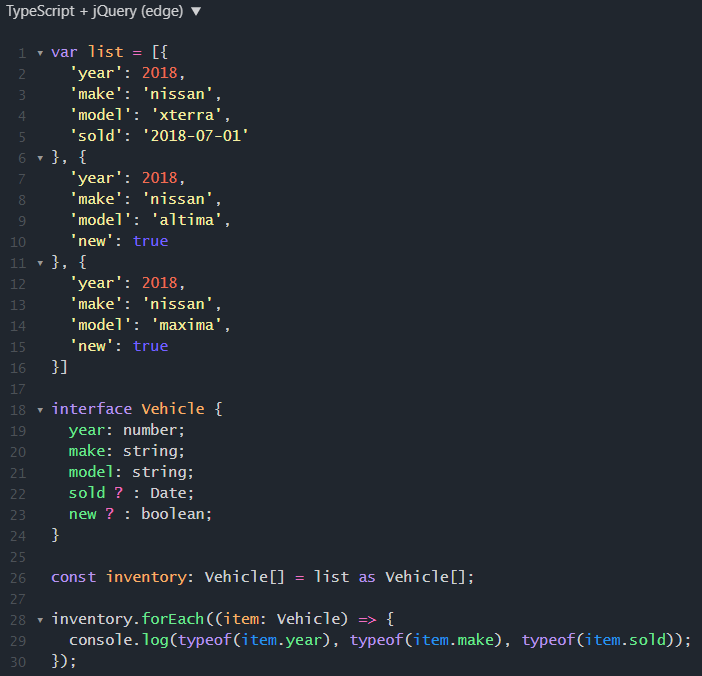
npm install --save ts-serializer
Build from Git
The library will be in the dist
folder
Usage
You start by importing the library decorators and the Serializable abstract class
In order to mark a class as serializable, you need to use the Serialize decorator and extend the abstract Serializable class.
The Serialize
decorator implements in the target class the serialize and deserialize methods required by the Serializable
class.
By default, the library does not serialize class properties if are not marked for serialization. In order to declare a property as serializable you use the SerializeProperty decorator.
After the class and the class properties are marked as serializable, you can use the serialize
and deserialize
methods and ts-serializer
will take care of things for you.
Full Example
For more information about the library check out serializer.dpopescu.me and ts-serializer Github page
During development, data comes from rest API in the format of JSON
format, So you need to write a code to convert the JSON
to interface
or class
in typescript.
This post talks about different ways of converting json to interface
We are going to learn various ways of converting JSON objects to Interface/class. In my previous article, Learned how to declared and implement typescript interfaces.This conversion is required to know as Front applications coded in typescript and calls REST API which interns calls backend services, and returns the response in JSON
format.
This JSON
object needs to transfer to interface/class to bind this data to UI.
Interfacs
have no implementation but it has some rules, where classes
or objects
that implement this interface should follow it.
How to Convert json to Object or interface
It is not required for the developer to perform anything to convert json to the interface; simply write code as per some rules to allow the typescript compiler to do the conversion.
First, Interface is defined with properties with same as JSON fields.
It is not required to match all fields in JSON object with interfaces, But
- Interface fields are a subset of JSON object
- Interface fields names and type should match with object data
We can do the conversion in two ways
- Implicit interface conversion
- Explicit interface conversion
Implicit interface conversion
The developer will not write any code to do the conversion. just follow guidelines as per above, Typescript compiler will do automatically
Here is an Example using Implicit conversion approach
The function returns Employee
data
Here are sequence of steps to follow
Typescript Deserialize Json To Class 8
- The
interface
should be created like as below usinginterface
keyword. - Declared interface with three properties, It is not compulsory to declared all properties. You can declare two fields also as per your requirement, but properties names should match with the name of JSON object keys
- Each
JSON
object representsEmployee
Object. if JSON object is list of arrays, Then in your app you should map it toArray
of employees orEmployee
[]
Here is an interface declared in Employee.ts
Explicit Interface conversion
In this, Developer will write a code to convert to interface.
This will be very useful when we need to customize or process some data before converting to interface/classes.
Using first-class functions - array’s method map(), which iterates JSON object array and do map the elements to the interface as seen in below code.
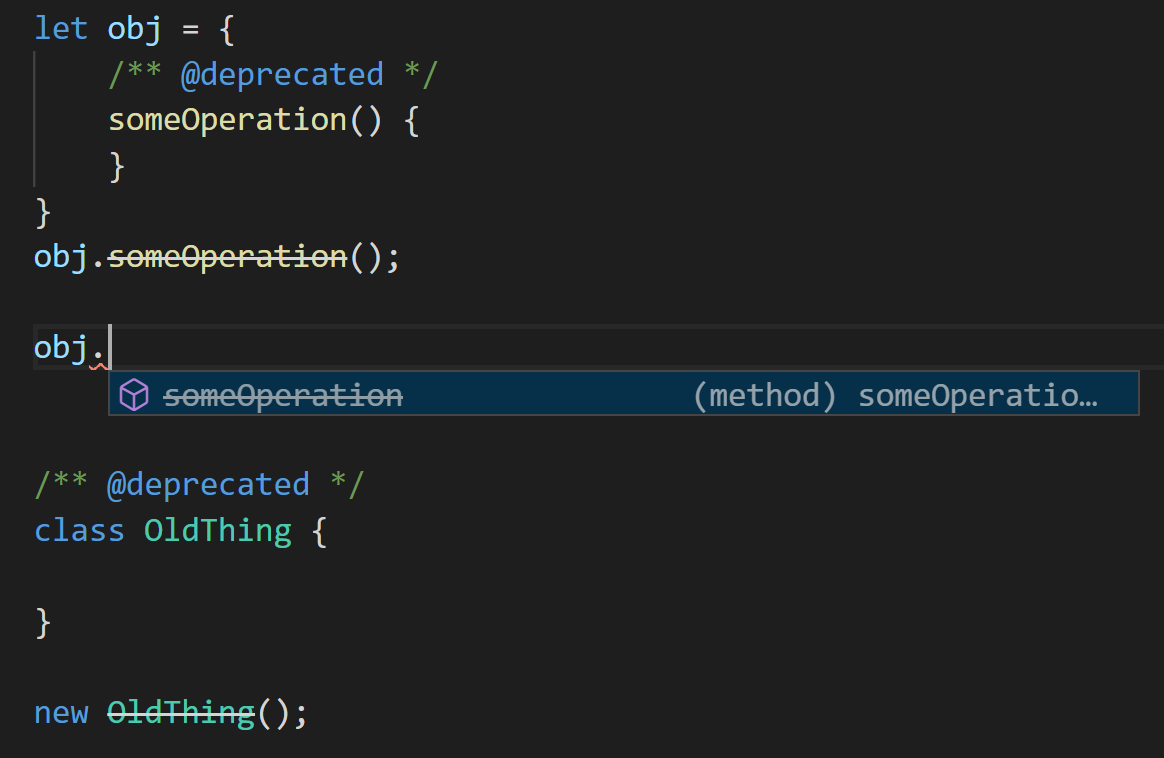
Nested JSON object interface mapping
In this example,
- Each
employee
hasaddress
with one to one mapping. - Created two interfaces one for
employee
and another foraddress
- declared mapping
address
in employee interface using like normal data type declaration.' - The same implicit interface mapping rules apply here to declare
address
interface.
This is way to we achieve map the custom JSON objects like recursive json
types or tree
model data. each parent contains children. We have seen all the above examples with interfaces, You can also do the map json to classes
Typescript Deserialize Json To Class 10
Summary
Typescript Deserialize Json To Class
To sum up,Discussed about different approaches to convert json to object or interface, Based on your convenience, you can chose one approach.